This section allows you to view all posts made by this member. Note that you can only see posts made in areas you currently have access to.
Topics - maverick
61
« on: July 03, 2017, 01:42:33 AM »
FUCK
62
« on: June 05, 2017, 02:54:09 AM »
Recommend me some interesting late night youtube videos to watch
63
« on: June 05, 2017, 01:32:02 AM »
Tired of going unrecognized on these forums.
64
« on: May 24, 2017, 08:29:06 PM »
first one to talk gets to stay on my aircraft
65
« on: May 23, 2017, 07:21:46 AM »
Stayed up all night making this beatable AI tic tac toe game. Spoiler #include <iostream> #include <stdlib.h> #include <cstdlib> #include <ctime>
using namespace std;
void MakeMove(char[]); void PrintBoard(char[]); void GameOver(char[]); void algorithm(char[]);
int main() { srand(time(0)); int choice; char mark; char board[10] = {'o', '1', '2', '3', '4', '5', '6', '7', '8', '9'}; MakeMove(board); }
void MakeMove(char board[]) { char choice; PrintBoard(board); cout << "\n Choose Square #: "; cin >> choice; cout << endl << endl; if (choice == '1' && board[1] == '1') { board[1] = 'X'; if (board[5] == '5') board[5] = 'O'; else algorithm(board); PrintBoard(board); GameOver(board); MakeMove(board); } else if (choice == '2' && board[2] == '2') { board[2] = 'X'; if (board[5] == '5') board[5] = 'O'; else algorithm(board); PrintBoard(board); GameOver(board); MakeMove(board); } else if (choice == '3' && board[3] == '3') { board[3] = 'X'; if (board[5] == '5') board[5] = 'O'; else algorithm(board); PrintBoard(board); GameOver(board); MakeMove(board); } else if (choice == '4' && board[4] == '4') { board[4] = 'X'; if (board[5] == '5') board[5] = 'O'; else algorithm(board); PrintBoard(board); GameOver(board); MakeMove(board); } else if (choice == '5' && board[5] == '5') { board[5] = 'X'; static int table[] = {1, 3, 7, 9}; int i = rand() % (sizeof table / sizeof *table); board[i] = 'O'; PrintBoard(board); GameOver(board); MakeMove(board); } else if (choice == '6' && board[6] == '6') { board[6] = 'X'; if (board[5] == '5') board[5] = 'O'; else algorithm(board); PrintBoard(board); GameOver(board); MakeMove(board); } else if (choice == '7' && board[7] == '7') { board[7] = 'X'; if (board[5] == '5') board[5] = 'O'; else algorithm(board); PrintBoard(board); GameOver(board); MakeMove(board); } else if (choice == '8' && board[8] == '8') { board[8] = 'X'; if (board[5] == '5') board[5] = 'O'; else algorithm(board); PrintBoard(board); GameOver(board); MakeMove(board); } else if (choice == '9' && board[9] == '9') { board[9] = 'X'; if (board[5] == '5') board[5] = 'O'; else algorithm(board); PrintBoard(board); GameOver(board); MakeMove(board); } else MakeMove(board); }
void PrintBoard(char b[]) { system("cls"); cout << "\n\n\t Tic Tac Toe\n\n"; cout << " Player 1 (X) - Player 2 (O)" << endl << endl; cout << "\t | | " <<endl; cout << "\t " << b[1] << " | " << b[2] << " | " << b[3] << " " <<endl; cout << "\t—--|—--|—--" <<endl; cout << "\t " << b[4] << " | " << b[5] << " | " << b[6] << " " <<endl; cout << "\t—--|—--|—--" <<endl; cout << "\t " << b[7] << " | " << b[8] << " | " << b[9] << " " <<endl; cout << "\t | | " <<endl << endl; }
void GameOver(char board[]) { if (board[1] != '1' && board[2] != '2' && board[3] != '3' && board[4] != '4' && board[5] != '5' && board[6] != '6' && board[7] != '7' && board[8] != '8' && board[9] != '9') { cout << "Cat's game!" << endl << endl; exit(0); } else if (board[1] == 'X' && board[2] == 'X' && board[3] == 'X') {cout << "You win!" << endl << endl; exit(0);} else if (board[1] == 'X' && board[4] == 'X' && board[7] == 'X') {cout << "You win!" << endl << endl; exit(0);} else if (board[2] == 'X' && board[5] == 'X' && board[8] == 'X') {cout << "You win!" << endl << endl; exit(0);} else if (board[4] == 'X' && board[5] == 'X' && board[6] == 'X') {cout << "You win!" << endl << endl; exit(0);} else if (board[3] == 'X' && board[6] == 'X' && board[9] == 'X') {cout << "You win!" << endl << endl; exit(0);} else if (board[7] == 'X' && board[8] == 'X' && board[9] == 'X') {cout << "You win!" << endl << endl; exit(0);} else if (board[1] == 'X' && board[5] == 'X' && board[9] == 'X') {cout << "You win!" << endl << endl; exit(0);} else if (board[3] == 'X' && board[5] == 'X' && board[7] == 'X') {cout << "You win!" << endl << endl; exit(0);} else if (board[1] == 'O' && board[2] == 'O' && board[3] == 'O') {cout << "You lose!" << endl << endl; exit(0);} else if (board[1] == 'O' && board[4] == 'O' && board[7] == 'O') {cout << "You lose!" << endl << endl; exit(0);} else if (board[2] == 'O' && board[5] == 'O' && board[8] == 'O') {cout << "You lose!" << endl << endl; exit(0);} else if (board[4] == 'O' && board[5] == 'O' && board[6] == 'O') {cout << "You lose!" << endl << endl; exit(0);} else if (board[3] == 'O' && board[6] == 'O' && board[9] == 'O') {cout << "You lose!" << endl << endl; exit(0);} else if (board[7] == 'O' && board[8] == 'O' && board[9] == 'O') {cout << "You lose!" << endl << endl; exit(0);} else if (board[1] == 'O' && board[5] == 'O' && board[9] == 'O') {cout << "You lose!" << endl << endl; exit(0);} else if (board[3] == 'O' && board[5] == 'O' && board[7] == 'O') {cout << "You lose!" << endl << endl; exit(0);} }
void algorithm(char board[]) { //offensive moves if (board[1] == 'O' && board[2] == 'O' && board[3] == '3') board[3] = 'O'; else if (board[1] == 'O' && board[4] == 'O' && board[7] == '7') board[7] = 'O'; else if (board[1] == 'O' && board[5] == 'O' && board[9] == '9') board[9] = 'O'; else if (board[1] == 'O' && board[3] == 'O' && board[2] == '2') board[2] = 'O'; else if (board[1] == 'O' && board[7] == 'O' && board[4] == '4') board[4] = 'O'; else if (board[1] == 'O' && board[9] == 'O' && board[5] == '5') board[5] = 'O'; else if (board[2] == 'O' && board[3] == 'O' && board[1] == '1') board[1] = 'O'; else if (board[2] == 'O' && board[5] == 'O' && board[8] == '8') board[8] = 'O'; else if (board[2] == 'O' && board[8] == 'O' && board[5] == '5') board[5] = 'O'; else if (board[3] == 'O' && board[6] == 'O' && board[9] == '9') board[9] = 'O'; else if (board[3] == 'O' && board[9] == 'O' && board[1] == '6') board[6] = 'O'; else if (board[3] == 'O' && board[5] == 'O' && board[7] == '7') board[7] = 'O'; //defensive moves else if (board[1] == 'X' && board[2] == 'X' && board[3] == '3') board[3] = 'O'; else if (board[1] == 'X' && board[4] == 'X' && board[7] == '7') board[7] = 'O'; else if (board[1] == 'X' && board[5] == 'X' && board[9] == '9') board[9] = 'O'; else if (board[1] == 'X' && board[3] == 'X' && board[2] == '2') board[2] = 'O'; else if (board[1] == 'X' && board[7] == 'X' && board[4] == '4') board[4] = 'O'; else if (board[1] == 'X' && board[9] == 'X' && board[5] == '5') board[5] = 'O'; else if (board[2] == 'X' && board[3] == 'X' && board[1] == '1') board[1] = 'O'; else if (board[2] == 'X' && board[5] == 'X' && board[8] == '8') board[8] = 'O'; else if (board[2] == 'X' && board[8] == 'X' && board[5] == '5') board[5] = 'O'; else if (board[3] == 'X' && board[6] == 'X' && board[9] == '9') board[9] = 'O'; else if (board[3] == 'X' && board[9] == 'X' && board[1] == '6') board[6] = 'O'; else if (board[3] == 'X' && board[5] == 'X' && board[7] == '7') board[7] = 'O'; //misc moves else if (board[1] == '1') board[1] = 'O'; else if (board[2] == '2') board[2] = 'O'; else if (board[3] == '3') board[3] = 'O'; else if (board[4] == '4') board[4] = 'O'; else if (board[5] == '5') board[5] = 'O'; else if (board[6] == '6') board[6] = 'O'; else if (board[7] == '7') board[7] = 'O'; else if (board[8] == '8') board[8] = 'O'; else if (board[9] == '9') board[9] = 'O'; }
Feel free to let me know how shitty it is.
66
« on: May 10, 2017, 08:15:35 PM »
please help
67
« on: April 11, 2017, 11:08:39 AM »
ian beat me to it
68
« on: April 10, 2017, 12:52:24 PM »
Figure some of you might be interested.
69
« on: March 26, 2017, 10:13:38 AM »
Been wanting to pick up Zelda but I don't have a current enough console. Since Switch is the newest one and there's not that much of a price difference, I should probably get that but I've been hearing there's all kinds of problems with it. What would you guys recommend?
70
« on: March 23, 2017, 10:14:48 PM »
?
71
« on: March 01, 2017, 03:11:14 PM »
72
« on: February 19, 2017, 03:38:21 PM »
73
« on: February 16, 2017, 03:33:26 PM »
in a perfect world everyone sees NASA and the US government for the decievers ththat they are, this is literal objective truth
74
« on: February 12, 2017, 08:06:15 PM »
?
75
« on: February 09, 2017, 04:05:21 PM »
AMA me anything
76
« on: February 08, 2017, 07:03:15 PM »
?
77
« on: February 02, 2017, 02:06:15 PM »
78
« on: December 26, 2016, 07:36:57 PM »
Need some ideas for good openers. I know you guys won't let me down.
Please discuss and thank you.
79
« on: December 09, 2016, 10:58:31 AM »
80
« on: November 27, 2016, 04:19:22 PM »
81
« on: November 25, 2016, 11:51:05 AM »
wtf was that shit
How do you go from DOFP to that
82
« on: November 11, 2016, 09:54:22 PM »
What did these geniuses expect when they put men & women together?
83
« on: November 03, 2016, 10:28:29 PM »
Very standard Marvel origin story. Not sure why some people seems to think it's one of the greats. It's everything you'd usually expect so people who love Marvel will love it and people who hate Marvel probably won't.
6/10
85
« on: October 22, 2016, 10:44:41 PM »
garbage team
86
« on: October 13, 2016, 10:40:08 AM »
87
« on: October 04, 2016, 10:28:52 PM »
Rank the Star Wars movies in order from favorite to least favorite and if you want add where you think Rogue One or any other future Star Wars movies is going to rank.
88
« on: September 24, 2016, 10:39:47 AM »
CNN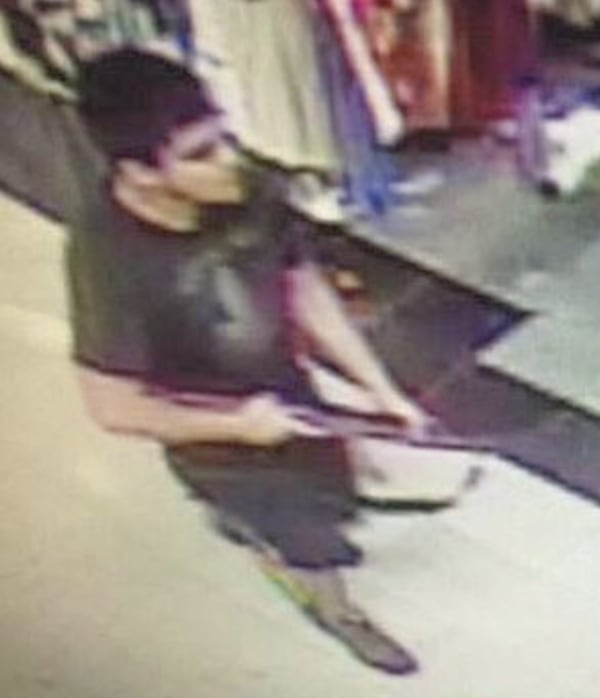 A man carrying a rifle entered a Macy's store at a mall in Washington state, shot dead four women and a man, and vanished into the night, police said.
Authorities believe only one person fired the shots Friday night at Cascade Mall in Burlington, about an hour north of Seattle.
89
« on: September 22, 2016, 09:31:02 AM »
90
« on: September 18, 2016, 10:27:36 PM »
Name somebody living that you can name in the same breath as them.
|